API references#
Main class#
The main class which computes brightness temperatures (Tb), mean radiating temperature (Tmr), and integrated absorption (Tau) for clear or cloudy conditions. Also returns all integrated quantities that the original TBMODEL, Cyber Version, returned ([Schroeder-Westwater-1991]).
|
Initialize TbCloudRTE |
Example:
Compute downwelling (rte.satellite == False
) brightness temperature for a typical Tropical Atmosphere.
from pyrtlib.tb_spectrum import TbCloudRTE
from pyrtlib.climatology import AtmosphericProfiles as atmp
from pyrtlib.utils import ppmv2gkg, mr2rh
z, p, _, t, md = atmp.gl_atm(atmp.TROPICAL)
gkg = ppmv2gkg(md[:, atmp.H2O], atmp.H2O)
rh = mr2rh(p, t, gkg)[0] / 100
ang = np.array([90.])
frq = np.arange(20, 201, 1)
rte = TbCloudRTE(z, p, t, rh, frq, ang)
rte.init_absmdl('R19SD')
rte.satellite = False
df = rte.execute()
df = df.set_index(frq)
df.tbtotal.plot(figsize=(12,8), xlabel="Frequency [GHz]", ylabel="Brightness Temperature [K]", grid=True)
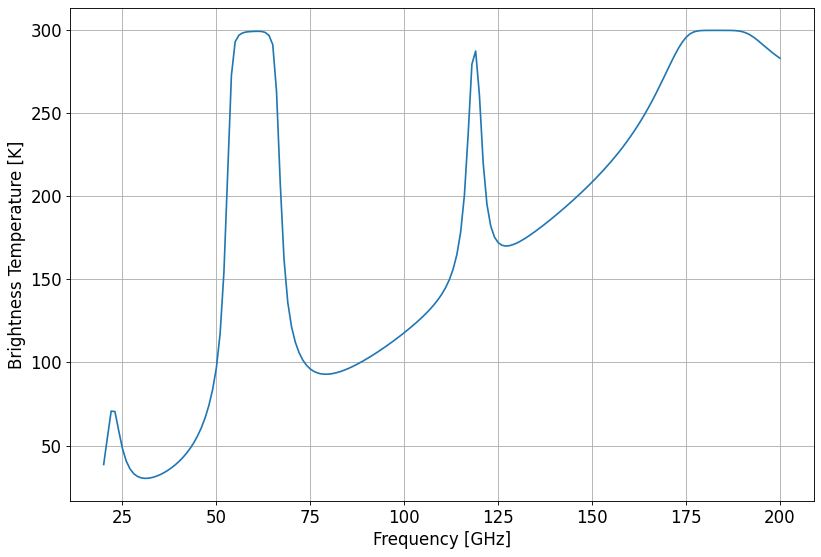
Also, it is possible to execute a combination of absorption models. The following example use R19SD
model for \(O_2\) and
R16
for \(H_2O\): to compute upwelling brightness temperature using emissivity surface.
from pyrtlib.tb_spectrum import TbCloudRTE
from pyrtlib.absorption_model import H2OAbsModel
from pyrtlib.climatology import AtmosphericProfiles as atmp
from pyrtlib.utils import ppmv2gkg, mr2rh
z, p, _, t, md = atmp.gl_atm(atmp.TROPICAL)
gkg = ppmv2gkg(md[:, atmp.H2O], atmp.H2O)
rh = mr2rh(p, t, gkg)[0] / 100
ang = np.array([90.])
frq = np.arange(20, 201, 1)
rte = TbCloudRTE(z, p, t, rh, frq, ang)
rte.emissivity = 0.9
rte.init_absmdl('R19SD')
H2OAbsModel.model = 'R16'
H2OAbsModel.set_ll()
df = rte.execute()
df = df.set_index(frq)
df.tbtotal.plot(figsize=(12,8), xlabel="Frequency [GHz]", ylabel="Brightness Temperature [K]", grid=True)
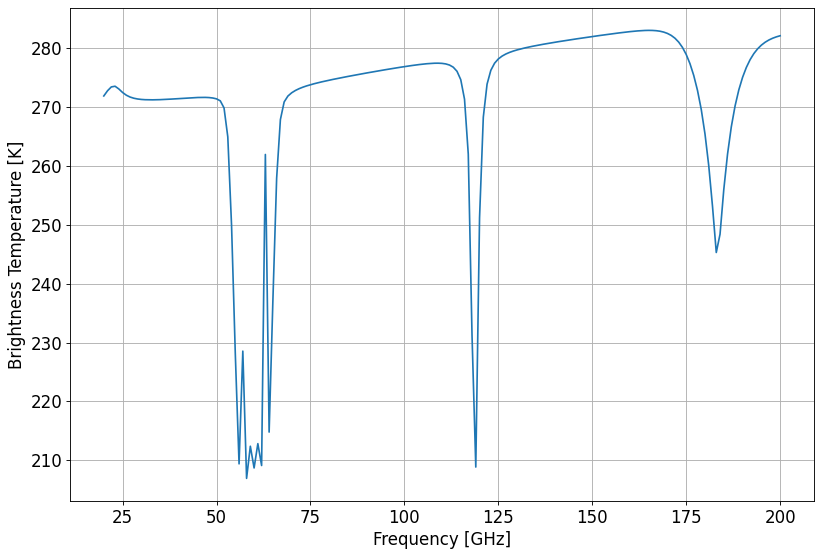
Standard Atmospheric Profiles#
Atmospheric constituent profiles (0-120km) (suplimented with other data) [ANDERSON] This file was partly copied from FASCOD2 routine MLATMB 10/11/87
The file contains 6 model profiles:
Model 1. Tropical
Model 2. Midlatitude Summer
Model 3. Midlatitude Winter
Model 4. Subarctic Summer
Model 5. Subarctic Winter
Model 6. U.S. Standard
Each of these profile contains data at 50 atmospheric levels: Altitude (km), Pressure (mb), Density (cm-3), Molec. densities (ppmv): 1(\(H_2O\)), 2(\(CO_2\)), 3(\(O_3\)), 4(\(N_2O\)), 5(\(CO\)), 6(\(CH_4\)), 7(\(O_2\)) Plus suplimental profiles where available.
AFGL Atmospheric Constituent Profiles (0-120km) |
|
Example:
from pyrtlib.climatology import AtmosphericProfiles as atmp
z, p, d, t, md = atmp.gl_atm(atmp.TROPICAL)
# index of available profiles
atmp.atm_profiles()
{0: 'Tropical',
1: 'Midlatitude Summer',
2: 'Midlatitude Winter',
3: 'Subarctic Summer',
4: 'Subarctic Winter',
5: 'US Standard'}
Radiative Transfer Equation#
RTE functions called from pyrtlib.rt_equation.RTEquation
:
bright
= compute temperature for the modified Planck radiancecloudy_absorption
= computes cloud (liquid and ice) absorption profilescloud_integrated_density
= integrates cloud water density of path ds (linear)cloud_radiating_temperature
= computes mean radiating temperature of a cloudclearsky_absorption
= computes clear-sky (\(H_2O\) and \(O_2\)) absorption profilesexponential_integration
= integrates (ln) absorption over profile layersplanck
= computes modified planck radiance and related quantitiesray_tracing
= computes refracted path length between profile levelsrefractivity
= computes vapor pressure and refractivity profilesvapor
= computes vapor pressure and vapor density
This class contains the main Radiative Transfer Equation functions. |
Absorption Models#
Computes absorption coefficient in atmosphere due to water vapor (\(H_2O\)), oxygen in air (\(O_2\)), ozone in air (\(O_3\)), suspended cloud liquid water droplets and collision-induced power absorption coefficient (neper/km) in air (“dry continuum”, mostly due to \(N_2\)-\(N_2\), but also contributions from \(O_2\)-\(N_2\) and \(O_2\)-\(O_2\))
This is an abstraction class to define the absorption model. |
|
This class contains the \(H_2O\) absorption model used in pyrtlib. |
|
This class contains the \(O_2\) absorption model used in pyrtlib. |
|
This class contains the \(O_3\) absorption model used in pyrtlib. |
|
This class contains the absorption model used in pyrtlib. |
|
This class contains the absorption model used in pyrtlib. |
To get all implemented models use the following code:
from pyrtlib.absorption_model import AbsModel
AbsModel.implemented_models()
['R98',
'R03',
'R16',
'R17',
'R19',
'R19SD',
'R20',
'R20SD',
'R21SD',
'R22SD']
Utility Functions#
The utils module contains funtions of general utility used in multiple places throughout pyrtlib.
This module contains the utils functions. |
Uncertainty#
This module has some tool to compute the absorption model sensitivity to the uncertainty of spectroscopic parameters, with the purpose of identifying the most significant contributions to the total uncertainty of modeled upwelling/downwelling brightness temperture.
This module provides the uncertainties affecting absorption model coefficients found in the litterature. |
|
Absorption model uncertainties for the spectroscopic parameters |
API Web Services#
Observations dataset web services which may be used in pyrtlib. Available datasets are the Wyoming Upper Air Archive (University of Wyoming), NCEI’s Integrated Radiosonde Archive version 2 (IGRA2) or the ERA5 Reanalysis model data (Copernicus Climate Change Service). See examples to get started to use these services.
Note
Parts of the code have been reused from the Siphon library.
Download and parse data from the University of Wyoming's upper air archive. |
|
Download and parse data from NCEI's Integrated Radiosonde Archive version 2. |
|
Read and Download data from ERA5 CDS Reanalysis model data |